What is enumerate in Python?
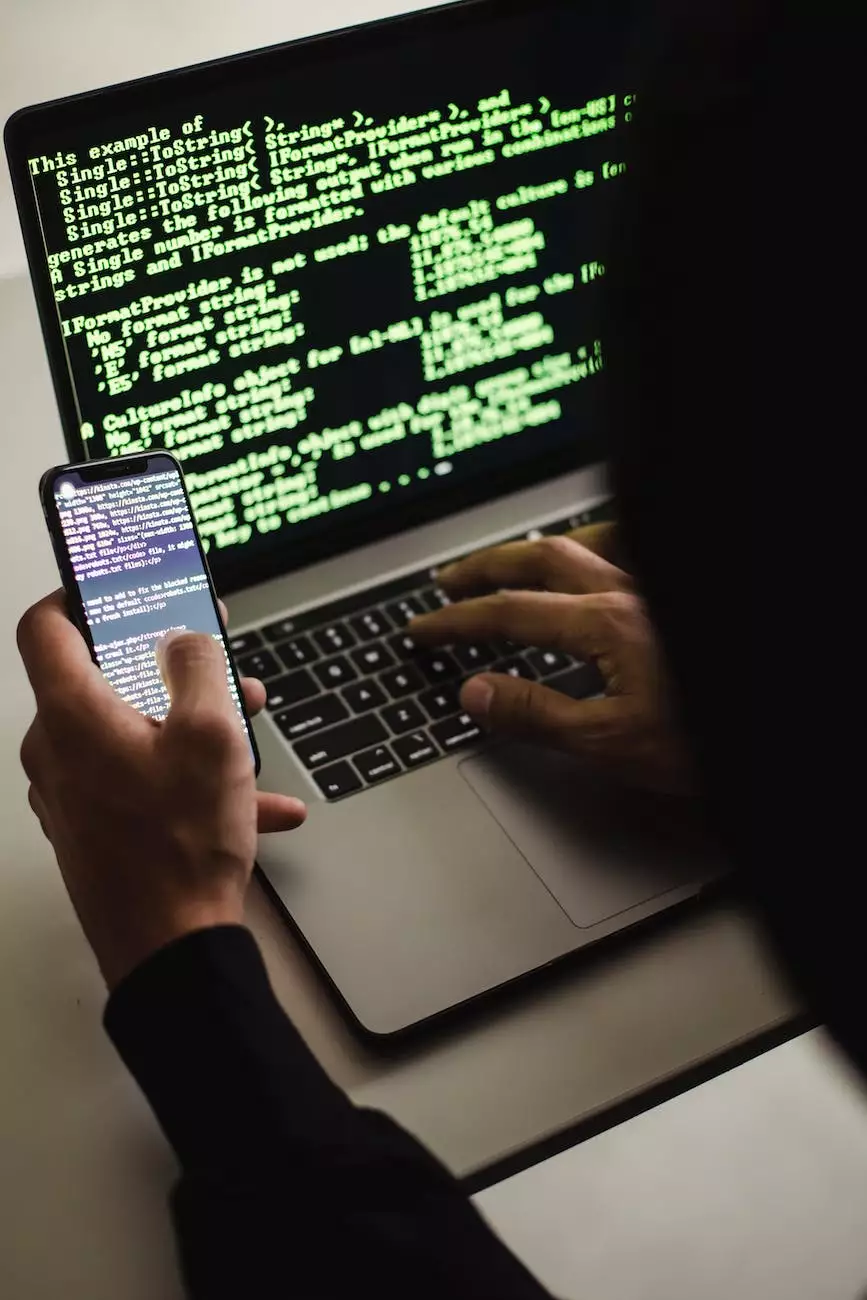
In the world of Python programming, the enumerate function plays a crucial role in improving code efficiency and readability. It offers a powerful way to loop over iterable objects while simultaneously keeping track of the current iteration index or position. By incorporating the enumerate function, you can greatly enhance your Python programming skills and simplify complex tasks. In this article, provided by Smartbiz Design - a leading provider of Business and Consumer Services in the field of Digital Marketing, we will delve into the workings of the enumerate function in Python and explore how it can be utilized effectively.
Understanding the Basics of enumerate
To grasp the power of the enumerate function, it is essential to understand its basic syntax:
enumerate(iterable, start=0)The enumerate function takes two parameters:
- iterable - an object that can be iterated over, such as a list, tuple, string, or dictionary.
- start (optional) - an integer that represents the start value of the index. By default, it is set to 0, but you can change it to any value as per your requirement.
The enumerate function returns an enumerate object that generates pairs of elements along with their corresponding indices. These pairs are accessible through iteration or can be converted into other data structures for further manipulation.
Utilizing enumerate in Practical Scenarios
Now that we have a basic understanding of the enumerate function, let's explore some practical use cases to showcase how it can be effectively employed:
1. Iterating Over a List
One of the most common use cases for enumerate is iterating over a list:
fruits = ['apple', 'banana', 'cherry'] for index, fruit in enumerate(fruits): print(f"Index: {index}, Fruit: {fruit}")Output:
Index: 0, Fruit: apple Index: 1, Fruit: banana Index: 2, Fruit: cherryIn the above example, the enumerate(fruits) function allows us to access both the index and the corresponding element of each item within the list fruits. This can be particularly useful when the order of elements matters.
2. Enumerating Strings
The enumerate function is not limited to just lists. It can also be effectively applied to iterate over strings:
message = "Hello, World!" for index, character in enumerate(message): print(f"Index: {index}, Character: {character}")Output:
Index: 0, Character: H Index: 1, Character: e Index: 2, Character: l Index: 3, Character: l Index: 4, Character: o Index: 5, Character: , Index: 6, Character: Index: 7, Character: W Index: 8, Character: o Index: 9, Character: r Index: 10, Character: l Index: 11, Character: d Index: 12, Character: !As you can see, the enumerate(message) function allows us to iterate over the characters in the string message while providing the corresponding indices.
3. Enumerating Dictionaries
In addition to lists and strings, the enumerate function can be employed with dictionaries as well. Although dictionaries are unordered, the enumerate function allows us to iterate over their items in a meaningful way:
company = {'name': 'Smartbiz Design', 'industry': 'Digital Marketing', 'location': 'California'} for index, key in enumerate(company.keys()): value = company[key] print(f"Index: {index}, Key: {key}, Value: {value}")Output:
Index: 0, Key: name, Value: Smartbiz Design Index: 1, Key: industry, Value: Digital Marketing Index: 2, Key: location, Value: CaliforniaBy using enumerate(company.keys()), we can access the keys of the dictionary company and obtain their corresponding values. This can be beneficial when you need to perform operations on both the keys and values of a dictionary.
Conclusion
The enumerate function in Python offers a convenient way to iterate over iterable objects while maintaining the index or position of each element. Through this article, we have explored the fundamentals of the enumerate function and various practical scenarios where it can be effectively applied. By leveraging the power of the enumerate function, you can enhance both the efficiency and readability of your Python code. Stay tuned to Smartbiz Design's Octal IT Solution Blog for more insights and tips on Python programming and digital marketing!